Data Structures and Algorithms Interview Questions With Answers
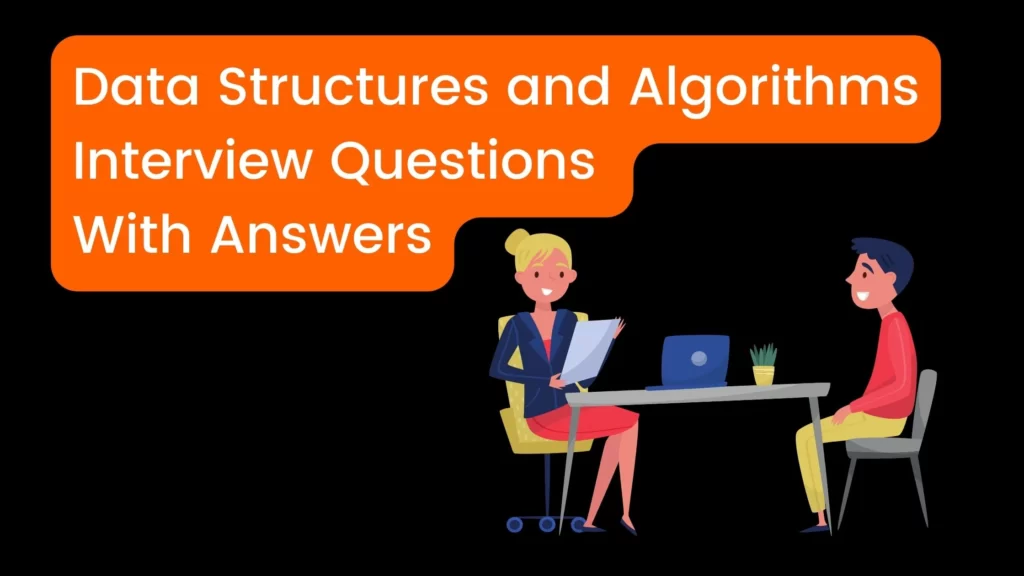
List of Data Structures and Algorithms Interview Questions With Answers
Table of Contents
What is Data Structure? Explain.
- The data structure is a way that specifies how to organize and manipulate the data. It also defines the relationship between them.
- Data Structures are the central part of many computer science algorithms as they enable the programmers to handle the data in an efficient way
What is a Stack?
Stack is an ordered list where insertion and deletion can only be done at one end called top. It is a recursive data structure having a pointer to its top element. The stack is sometimes called a Last-In-First-Out (LIFO) list i.e. the element which is inserted first in the stack will be deleted last from the stack.
What is the difference between file structure and storage structure?
- Storage structure: It represents the data structure in the computer memory.
- File structure: It is a representation of the sub-memory storage structure.
Which data structure is used to perform recursion?
The stack data structure is used in recursion due to its last in first out nature. The operating system maintains the stack to save the iteration variables at each function call.
List the area of applications of Data Structure?
- Compiler Design
- Operating System
- Database Management System
- Statistical analysis package
- Numerical Analysis
- Graphics
- Artificial Intelligence
- Simulation
Can you tell how linear data structures differ from non-linear data structures?
- If the elements of a data structure result in a sequence or a linear list then it is called a linear data structure.
- Whereas, traversal of nodes happens in a non-linear fashion in non-linear data structures.
What is an array?
- Arrays are the collection of similar types of data stored at contiguous memory locations.
- It is the simplest data structure where the data element can be accessed randomly just by using its index number.
What is a linked list?
A linked list is a data structure that has a sequence of nodes where every node is connected to the next node using a reference pointer. The elements are not stored in adjacent memory locations. They are linked using pointers to form a chain. This forms a chain-like link for data storage.
Are linked lists of linear or non-linear types?
Linked lists can be considered both linear and non-linear data structures. This depends upon the application that they are used for.
- When the linked list is used for access strategies, it is considered as a linear data structure. When they are used for data storage, they can be considered as a non-linear data structure.
What are the applications of a Stack?
- Check for balanced parentheses in an expression
- Evaluation of a postfix expression
- The problem of Infix to postfix conversion
- Reverse a string
Conclusion
The questions in this article are a great place to start, but they should be considered as sample questions rather than the definitive list of interview questions you will face during your coding interview. Check out our complete guide to data structures and algorithms here!